Javascript Primer
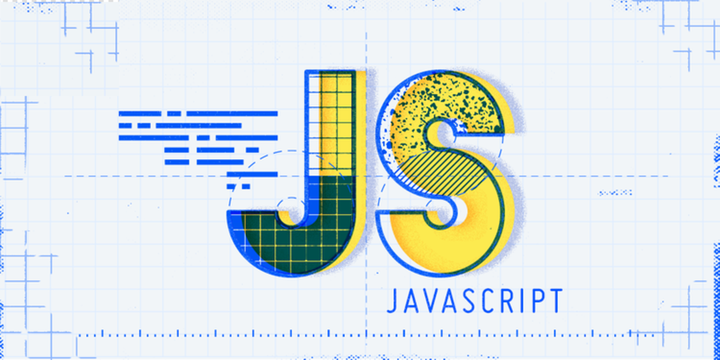
Using Functions as Arguments to Other Functions
Javascript functions can be treated as objects, which means we can use one function as the argument to another. For example,
function myFunc(nameFunction) {
return ("Hello "+nameFunction()+".");
}
console.log(myFunc(function() {
return "TechyOwls!";
}));
The myFunc
function defines a parameter called nameFunction
that invokes to get the
value to insert into the string it returns. We passed a function that returns TechyOwls!
as the argument to myFunc, which produces following output.
Hello TechyOwls!
Functions can be chained together, building up more complex functionality from small and easily tested pieces of code.
Let's see an example:
function myFunc(nameFunction) {
return ("Hello "+ nameFunction()+ ".");
}
function printName(nameFunction,printFunction) {
printFunction(myFunc(nameFunction));
}
printName(function() {return "TechyOwls!"}, console.log)
Above example produces the following output:
Hello TechyOwls!
Using Arrow Functions
Arrow functions- also knows as fat arrow functions or lambda expressions are an alternative way of defining functions and are often used to define functions that are used only as arguments to other functions.
const myFunc= (nameFunction) => ("Hello "+nameFunction()+".");
const printName= (nameFunction,printFunction) => printFunction(myFunc(nameFunction));
printName(function() { return "TechyOwls!"},console.log);
Above code performs the same works as the ones used in Using Functions as Arguments to Other Functions . There are three parts to an arrow function:
- Input parameters
- An equal sign and a greater-than sign (the “arrow”)
- And finally the function result.
The return keyword and curly braces are required only if the arrow function needs to execute more than one statement.
Using VARIABLE CLOSURE
If we define a function inside another function–creating inner and outer functions– then the inner function is able to access the outer function's variable, using a feature called closure, like
function myFunc(name) {
let myLocalVariable="Cloudy";
let innerFunction= function() {
return ("Hello "+name+". Today is"+ myLocalVariable+".");
};
return innerFunction();
}
console.log(myFunc("TechyOwls"));
Hello TechyOwls. Today is Cloudy
The inner function in above example is able to access the local variable of the outer function, including it's parameter.
This is a powerful feature that means we don't have to define parameters on inner functions to pass around data values,
but caution
is required, because it is easy to get unexpected result when using common variable names
like counter or index, where we may not realize that we are reusing a variable name from the outer function.
Working with Strings
JavaScript provides string objects with a basic set of properties and methods, some of the most useful of which are described bellow:
charAt(index)
The charAt(index) method returns the character at the specified index in a string. The index of the first character is 0, the second character is 1, and so on.
const message="Hello TechyOwls!";
console.log(message.charAt(0))
output: H
Tip: The index of the last character in a string is string.length-1, the second last character is string.length-2, and so on (See “More Examples”).
concat(string)
The concat() method is used to join two or more strings. This method does not change the existing strings, but returns a new string containing the text of the joined strings.
const str1 = "Hello ";
const str2 = "world!";
const res = str1.concat(str2);
output: "Hello world!"
indexOf(term, start)
The indexOf() method returns the index within the calling String object of the first occurrence of the specified value, starting the search at fromIndex. Returns -1 if the value is not found.
const paragraph = 'The quick brown fox jumps over the lazy dog. If the dog barked, was it really lazy?';
const searchTerm = 'dog';
const indexOfFirst = paragraph.indexOf(searchTerm);
console.log('The index of the first "' + searchTerm + '" from the beginning is ' + indexOfFirst);
// expected output: "The index of the first "dog" from the beginning is 40"
console.log('The index of the 2nd "' + searchTerm + '" is ' + paragraph.indexOf(searchTerm, (indexOfFirst + 1)));
// expected output: "The index of the 2nd "dog" is 52"
Using the Spread Operator
The spread operator is used to expand an array so that its contents can be used as function arguments.
Let's define a function that accepts multiple arguments and then invokes it using the values in an array with and without the spread operator.
function printItems(numValue, stringValue, boolValue) {
console.log(`Number: ${numValue}`);
console.log(`String: ${stringValue}`);
console.log(`Boolean: ${boolValue}`);
}
let myArray = [100, "Adam", true];
printItems(myArray[0], myArray[1], myArray[2]);
printItems(...myArray);
The spread operator is an ellipsis (a sequence of three periods), and it causes the array to be unpacked and passed to the printItems function as individual arguments. The spread operator also makes it easy to concatenate arrays. Let's see an example
let myArray = [100, "Adam", true];
let myOtherArray = [200, "Bob", false, ...myArray];
myOtherArray.forEach((value, index) => console.log(`Index ${index}: ${value}`));
Index 0: 200
Index 1: Bob
Index 2: false
Index 3: 100
Index 4: Adam
Index 5: true